User Guide¶
This user guide to pyAPP7 is structured into four parts. First, an overview over the package strcuture is provided. The second section describes how to read and write APP files (aircraft, missions and performance charts) using Python. The last two sections describe how to execute APP’s mission computations and performance chart computations by using Python and parse the results written by APP.
Package Structure¶
The pyAPP7 package comprises the following modules:
- Files
Classes for Reading and Writing APP Files.
- Datatypes
Classes defining APP sepcific, custom data types
- Mission
Classes for executing Mission Computations and reading the results.
- Performance
Classes for executing Performance Charts computations and reading the results.
- Database
Helper class to read APP’s string table.
- Global
Constants as used in APP.
- Units
Unit conversion factors as used in APP.
Reading and Writing APP Files¶
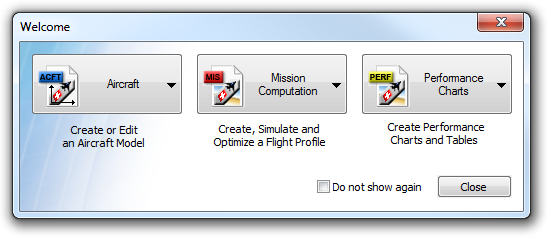
For each APP7 filetype, pyAPP7 offers a class to read, manipulate and write a file. The classes are located in the Files module:
APP File |
pyAPP7 Class |
---|---|
Aircraft (.acft) |
Files.AircraftModel |
Mission Computation (.mis) |
Files.MissionComputationFile |
Performance Charts (.perf) |
Files.PerformanceChartFile |
The classes are located in the Files module:
-
class
pyAPP7.Files.
AircraftModel
Holds the APP7 aircraft model that is used to read and write APP .acft files
Each type of data (Mass&Limits, Aerodynamcis, Propulsion, Stores) is stored in two lists: one list containing names and one list containing data. These two lists have to have the same length. The configurations are built by using these list indices. Take proper care when manipulating these lists manually and update the ‘ProjectAircraft’ (m_Prj).
Examples
The best way to create an instance of an AircraftModel is to use the classmethod fromFile:
from pyAPP7 import Files acft = Files.AircraftModel.fromFile(r'myAircraft.acft')
- Variables
m_GeneralData (GeneralData) – General data about the aircraft
text (Text) – Content of the comment text box in ‘General Data’
configName (list[str]) – list holding the names of the Mass&Limits datasets (‘Config’ classes)
aeroName (list[str]) – list holding the names of the Aerodynamics datasets (‘Aero’ classes)
propulsionName (list[str]) – list holding the names of the Propulsion datasets (‘PropulsionData’ child classes)
storeName (list[str]) – list holding the names of the Store datasets (‘Store’ classes)
m_config (list[Config]) – list of the Mass&Limits datasets (‘Config’ classes)
m_aero (list[Aero]) – list of the Aerodynamics datasets (‘Aero’ classes)
m_propulsion (list[PropulsionData]) – list of the Propulsion datasets (‘PropulsionData’ child classes)
m_store (list[Store]) – list of the Store datasets (‘Store’ classes)
m_Prj (ProjectAircraft) – Contains the Configurations and Store Configurations
-
class
pyAPP7.Files.
MissionComputationFile
Reads an APP .mis file
This class reads an APP mission computation file. Most of the data is stored in a ProjectAircraftSetting object (aircraft configuration and stores) and a MissionDefinition object (initial conditions, list of segments). When manipulating mission files, consult the source code and documentation of these two classes.
Note
Data for APP’s “Parameter Study” computation mode is read as well (into the variationData attribute). However, APP’s command line mode does not support this computation type
Examples
The best way to create an instance of a MissionComputationFile is to use the classmethod fromFile:
from pyAPP7 import Files mis = Files.MissionComputationFile.fromFile(r'myMission.mis')
- Variables
text (Text) – Description text
name (str) – name of the mission computation
author (str) – name of the author of the mission file
aircraftpath (str) – path to the aircraft, either relative (to the location of the mis file) or absolute
projectAircraftSetting (ProjectAircraftSetting) – holds the used configuration of the aircraft and settings of stores
misDef (MissionDefinition) – Holds the initial conditions and the list of segments
resData (ResArrayData) – Holds the computation type (CMP_MISSION or CMP_MISSIONVAR)
variationData (VariationData) – Holds data for the Parameter Study mission computation type
-
class
pyAPP7.Files.
PerformanceChartFile
Reads an APP .perf file
This class reads an APP performance chart file. Most of the data is stored in a ProjectAircraftSetting object (aircraft, configuration and stores), a FlightData object (initial conditions and flight state) and a PointPerfSolver child class object (specific data, related to the type of performance chart).
Note
Not all types of point performance charts can be computed by the APP command line mode. See documentation for valid types.
Examples
The best way to create an instance of a PerformanceChartFile is to use the classmethod fromFile:
from pyAPP7 import Files chart = Files.PerformanceChartFile.fromFile(r'myPerfFile.perf')
- Variables
text (Text) – Description text
name (str) – name of the mission computation
author (str) – name of the author of the mission file
aircraftpath (str) – path to the aircraft, either relative (to the location of the perf file) or absolute
projectAircraftSetting (ProjectAircraftSetting) – holds the used configuration of the aircraft and settings of stores
flightData (FlightData) – holds the flight state (initial conditions)
perf (PointPerfSolver) – instance of a child class of PointPerfSolver, defines the type of performance chart
NExtReal¶
APP defines two custom data types: NExtReal and XTables. When using pyAPP7 to manipulate APP files, it is important to understand these data types.
NExtReals are recognizable in the APP user interface by a text followed by a value and a unit:
-
class
pyAPP7.Datatypes.
NExtReal
APP datatype that wraps a float and allows to specify a label, type of variable (through an index string) and indicate if the value is a limiter
- Variables
xx (float) – value of variable
label (str) – label of the value, e.g. ‘[Mach]’
realIdx (str) – index (type) of variable, e.g. ‘REAL_MACH’
limitActive (int) – 0 or 1, depends on whether the variable has an active limit. E.g used for Max. Take-Off Mass
Note
use readASCIILimited and writeASCIILimited if the variable is a limited value.
Examples
When using pyAPP7 to read APP files, usually no direct use of this type is needed. This information is mostly for developers/maintainers. The text format of a simple, non-limited NExtReal looks like this:
[Mach] REAL_MACH 0.985
This is parsed using readASCII with the flag full=True. The full flag has to be set to True to read the index string ‘REAL_MACH’.
>>> val = NExtReal() >>> f = open('path to text file') >>> val.readASCII(f, full=True)
resulting in the following attributes:
val.xx = 0.985 val.realIdx = 'REAL_MACH' val.label = '[Mach]' val.limitActive = 0
If the text format has no index string,
[Mach] 0.985
readASCII is called with with the flag full=False:
>>> val = NExtReal() >>> f = open('path to text file') >>> val.readASCII(f)
XTable¶
XTables are used everywhere you see a spreadsheet-like table in APP. pyAPP7 uses the numpy module to store data tables. numpy offers a lot of functionality to manipulate arrays. pyAPP7 defines four different tables, with increasing dimensionality: X0Table, X1Table, X2Table and X3Table.
The X0Table is used for one-column data ranges, for example in performance charts for the X-Range and Parameter range.
-
class
pyAPP7.Datatypes.
X0Table
Holds a 1D table (data range)
- Variables
data (list[str]) – Table data with table factor and interpolation settings
table (ndarray) – numpy array of shape (N,1)
label (str) – Header string
X0Typ (str) – APP variable type
The X1Table is a simple two-column table. An example would be the Mach limit or CLmax table. The data is stored in a two-dimensional numpy array.
-
class
pyAPP7.Datatypes.
X1Table
Holds a 2D table
- Variables
data (list[str]) – Table data with table factor and interpolation settings
table (ndarray) – numpy array of shape (N,2)
label (str) – Header string
The X2Table is a list of two-column tables. An example would be the induced drag tables or the max. thrust tables. The data is stored in a list of two-dimensional numpy arrays (table attribute). Each table also has a value (for the induced drag table that would be a Mach number). The values are stored in the value list. The table and value list have the same length and same ordering.
-
class
pyAPP7.Datatypes.
X2Table
(embedded=False) Holds a list of 2D tables
- Variables
data (list[str]) – Table data with table factor and interpolation settings
table (list[ndarray]) – list of numpy arrays of shape (N,2)
value (list[float]) – value of each table
label (str) – Header string
embedded (bool) – True if table is embedded in an ‘X3Table’. Disables reading/writing of header (data and label)
The X3Table class is used in APP for the fuel flow table. The X3Table consists of a list of X2Tables and corresponding values.
-
class
pyAPP7.Datatypes.
X3Table
Holds a list of X2Tables.
This class holds a list of X2Tables and a value for each table.
- Variables
data (list[str]) – Table data with table factor and interpolation settings
table (list[X2Table]) – list of X2Table instances
value (list[float]) – value of each table
label (str) – Header string
Variables¶
When executing APP via the command line, the user can specify what variables will be written in the output result file. By default, pyAPP7 uses the included ParameterList_All.par file to specify the variables. The ouput data is stored in a large numpy table, and the variable can be best accessed by it’s index. The following table presents the current mapping of indices to the variables when using the default parameter list file.
Index |
Variable Name |
(SI) |
(British) |
0 |
Acceleration |
[m/sec2] |
[ft/sec2] |
1 |
SEP (Accel) |
[m/sec2] |
[KTS/s] |
2 |
X-Acc. |
[m/sec2] |
[ft/sec2] |
3 |
Z-Acc. |
[m/sec2] |
[ft/sec2] |
4 |
Advance Ratio |
[-] |
[-] |
5 |
Altitude |
[m] |
[ft] |
6 |
AoA |
[deg] |
[deg] |
7 |
Attitude |
[deg] |
[deg] |
8 |
Battery Energy |
[kJ] |
[Wh] |
9 |
Battery SOC |
[%] |
[%] |
10 |
Propeller Beta |
[deg] |
[deg] |
11 |
CAS |
[m/sec] |
[nm/hr] |
12 |
CD |
[-] |
[-] |
13 |
CD0 |
[-] |
[-] |
14 |
CDi |
[-] |
[-] |
15 |
CDs |
[-] |
[-] |
16 |
CL |
[-] |
[-] |
17 |
CL/CD |
[-] |
[-] |
18 |
CLmax |
[-] |
[-] |
19 |
CO2 Mass |
[kg] |
[lbs] |
20 |
Thrust cos(AoA+sigma) |
[N] |
[lbf] |
21 |
CP |
[-] |
[-] |
22 |
(M/SFC)(L/D) |
[-] |
[-] |
23 |
CT |
[-] |
[-] |
24 |
Density |
[kg/m3] |
[slug/ft3] |
25 |
Distance |
[km] |
[nm] |
26 |
Drag |
[N] |
[lbf] |
27 |
Drag Area |
[m2] |
[ft2] |
28 |
dT |
[K] |
[K] |
29 |
EAS |
[m/sec] |
[nm/hr] |
30 |
Energy Height |
[m] |
[ft] |
31 |
Ekin |
[Nm] |
[lbf ft] |
32 |
Epot |
[Nm] |
[lbf ft] |
33 |
Energy Specific Range |
[m/J] |
[m/J] |
34 |
Etot |
[Nm] |
[lbf ft] |
35 |
Friction Force |
[N] |
[lbf] |
36 |
Fuel Flow |
[kg/sec] |
[lbs/hr] |
37 |
Fuel Mass |
[kg] |
[lbs] |
38 |
Fuel Percent |
[%] |
[%] |
39 |
Fuel Percent (Internal) |
[%] |
[%] |
40 |
Climb Angle |
[deg] |
[deg] |
41 |
Generator Power |
[%] |
[%] |
42 |
Ground Force |
[N] |
[lbf] |
43 |
Load Factor |
[-] |
[-] |
44 |
Lift |
[N] |
[lbf] |
45 |
Lift Area |
[m2] |
[ft2] |
46 |
Placard Mach |
[-] |
[-] |
47 |
Mach |
[-] |
[-] |
48 |
Mass |
[kg] |
[lbs] |
49 |
Max. Thrust |
[N] |
[lbf] |
50 |
Min. Thrust |
[N] |
[lbf] |
51 |
Motor Eta |
[%] |
[%] |
52 |
Motor Torque |
[Nm] |
[lbf ft] |
53 |
Payload |
[%] |
[%] |
54 |
Power Setting |
[%] |
[%] |
55 |
Power Consumption |
[W] |
[W] |
56 |
Power Required |
[W] |
[shp] |
57 |
Pull-Up Rate |
[deg/sec] |
[deg/sec] |
58 |
Pressure Altitude |
[m] |
[ft] |
59 |
Pressure |
[N/m2] |
[lbf/ft2] |
60 |
Propeller Efficiency |
[%] |
[%] |
61 |
Dynamic Pressure |
[N/m2] |
[lbf/ft2] |
62 |
Engine Revolution |
[rpm] |
[rpm] |
63 |
Seg. CO2 Mass |
[kg] |
[lbs] |
64 |
Seg. Dist. |
[km] |
[nm] |
65 |
Seg. Fuel |
[kg] |
[lbs] |
66 |
Seg. Time |
[min] |
[min] |
67 |
SEP |
[m/sec] |
[ft/sec] |
68 |
Configuration Nr. |
[-] |
[-] |
69 |
SFC |
[kg/(sec N)] |
[lbs/(hr lbf)] |
70 |
Shaft Power |
[W] |
[shp] |
71 |
Speed of Sound |
[m/sec] |
[ft/sec] |
72 |
Specific Range |
[km/kg] |
[nm/lbs] |
73 |
Reference Area |
[m2] |
[ft2] |
74 |
TAS |
[m/sec] |
[nm/hr] |
75 |
Temperature |
[K] |
[K] |
76 |
Thrust |
[N] |
[lbf] |
77 |
Time |
[sec] |
[sec] |
78 |
Turn Radius |
[m] |
[ft] |
79 |
Turn Rate |
[deg/sec] |
[deg/sec] |
80 |
T/Tmax |
[-] |
[-] |
81 |
Turns |
[turn] |
[turn] |
82 |
Velocity |
[m/sec] |
[nm/hr] |
83 |
Minimum Unstick Speed |
[m/sec] |
[nm/hr] |
84 |
Minimum Unstick Speed (CAS) |
[m/sec] |
[nm/hr] |
85 |
Stall Speed |
[m/sec] |
[nm/hr] |
86 |
Stall Speed (CAS) |
[m/sec] |
[nm/hr] |
87 |
Vx |
[m/sec] |
[nm/hr] |
88 |
Climb Speed |
[m/sec] |
[ft/sec] |
Mission Computations¶
The Mission module, specifically the class MissionComputation, is used to run the APP command line mode for mission computations and parse the result text file.
-
class
pyAPP7.Mission.
MissionComputation
(APP7Directory='C:\\Program Files (x86)\\ALR Aerospace\\APP 7 Professional Edition') Class to execute APP and subsequently load the results.
This class is a helper class to execute APP mission computations. After creating an instance of this object, execute the ‘run’ function. The result will be loaded into the ‘result’ attribute. ‘result’ is of type MissionResult, see the documentation of the MissionResult class for further details.
Note
The ‘Parameter Study’ computation type can not be computed with the APP command line mode.
Examples
This example shows how to run a mission computation and obtain an instance of the mission result:
from pyAPP7 import Mission misCmp = Mission.MissionComputation() misCmp.run(r'myMission.mis') result = misCmp.getResult()
This example assumes APP is installed in the default directory.
- Variables
output (str) – Path to the text file with the mission results written by APP
result (MissionResult) – The result of the mission computation, parsed from the ‘output’ text file
misCompFile (Files.MissionComputationFile) – Instance of a MissionComputationFile (APP .mis file). Is available once the method run was called
db (Database) – Instance of a Database object
inputfile (str) – Path to the APP mis file
A result from an APP command line computation can also be directly read by using the MissionResult class.
-
class
pyAPP7.Mission.
MissionResult
This class can read the APP mission result text file.
Examples
This example shows how to read a mission result directly from a text file. This is useful to read results from past mission computations, for example when conduction batch simulations:
from pyAPP7 import Mission res = Mission.MissionResult.fromFile(r'myMission.mis_ouput.txt')
- Variables
output (dict) – Dictionary containing the mission flags, error text, number- and list of variables
segments (list[MissionResultSegment]) – A list of MissionResultSegment class instances, holding the results of each segment
initialSettings (MissionResultSegment()) – The initial settings of the mission
Performance Charts¶
The Performance module, specifically the class PerformanceChart, is used to run the APP command line mode for performance chart computations and parse the result text file.
-
class
pyAPP7.Performance.
PerformanceChart
(APP7Directory='C:\\Program Files (x86)\\ALR Aerospace\\APP 7 Professional Edition') Helper class to execute a performance chart computation from an existing .perf file.
- Variables
inputfile (str) – Path to the input .perf file
perfFile (PerformanceChartFile) – Parsed input APP7 .perf file
output (str) – Path to the resulting txt file
result (PerformanceChartResult) – Result
APP7Path (str) – Full path to the APP7 executable
- Parameters
APP7Directory (str, optional) – Path to the location of the APP7 executable.
- Raises
ValueError – If the APP7 executable is not found in the specified directory
A result from an APP command line computation can also be directly read by using the PerformanceChartResult class.
-
class
pyAPP7.Performance.
PerformanceChartResult
Reads a result txt file written by the APP7 command line mode for a performance chart.
Examples
This example shows how to read a performance chart result directly from a text file. This is useful to read results from past computations, for example when conduction batch computations:
from pyAPP7 import Performance res = Performance.PerformanceChartResult.fromFile(r'myChart.perf_ouput.txt')
- Variables
output (dict) – stores the result meta-data
lines (List[ResultLine]) – holds the data of each line of a performanc chart